CSS variables allow us to put information in a common location. We can then use that information in multiple places in our CSS.
It works really well for things like colours and fonts. We define them once, at the top, then use them in multiple places. It’s especially helpful for colours because we don’t have to remember the hex value, just the name of the variable—which our code editor should autocomplete for us.
We can define our variables anywhere in the CSS, but it’s often best to define them at the very top, in the :root
selector.
:root {
--color-primary: red;
--border-radius: 4px;
}
html {
font-family: sans-serif;
}
.card {
max-width: 24em;
border: 3px solid var(--color-primary);
border-radius: var(--border-radius);
}
If everything was spelled properly, it should still look exactly the same in the browser:
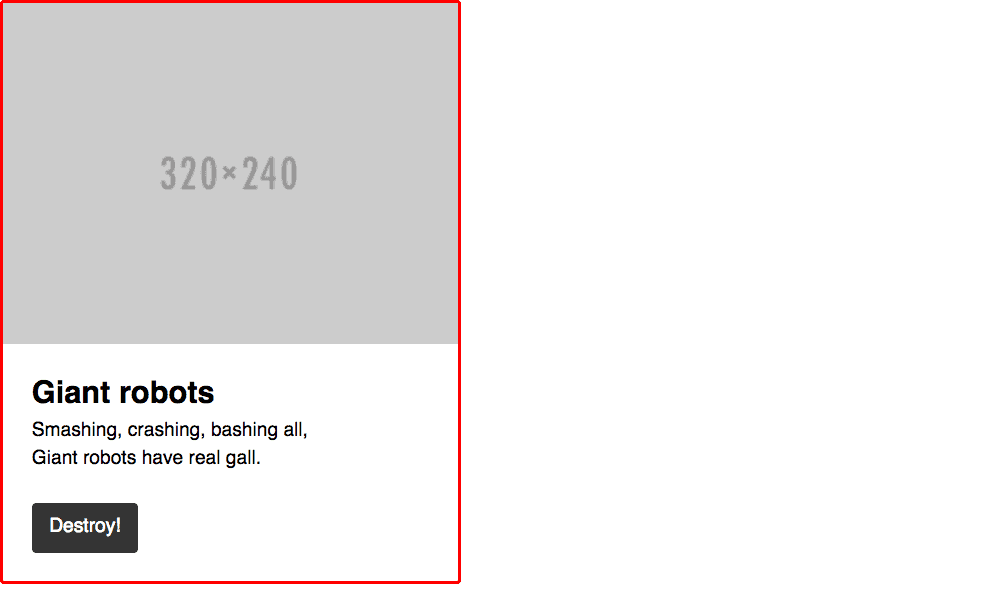